处理 MAPDL Full 文件 (.full)#
MAPDL full 文件是一个 FORTRAN 格式的二进制文件,包含来自 Ansys 分析的质量和刚度。使用 Pyansys 可以将其作为稀疏矩阵或全矩阵加载到内存中。
Reading a Full File#
本例读入与上例相关的质量和刚度矩阵。 load_km
对自由度进行排序,节点从最小到最大排序,每个自由度(即 X、Y、Z)在每个节点内排序。
默认情况下,矩阵 k
和 m
是稀疏的,但如果没有安装 scipy
,或者使用了可选参数 as_sparse=False
,那么它们将是完整的 numpy 数组。
默认情况下, load_km
输出两个矩阵的上三角。可以通过访问 fobj.const
来识别分析的受约束节点,其中受约束的自由度为 True ,所有其他自由度为 False 。
这与 dof_ref
中的参照度相对应。
默认情况下,dof_ref 是不排序的。要对这些值进行排序,请设置 sort==True
。本例中启用排序是为了稍后绘制数值。
from ansys.mapdl import reader as pymapdl_reader
from ansys.mapdl.reader import examples
# 创建 result reader 对象并读入 full 文件
full = pymapdl_reader.read_binary(examples.fullfile)
dof_ref, k, m = full.load_km(sort=True)
ANSYS 只在 full 文件中存储上三角矩阵。要创建全矩阵,请执行以下操作:
k += sparse.triu(k, 1).T
m += sparse.triu(m, 1).T
如果安装了 scipy
,就可以求解系统的固有频率和模态振型。
import numpy as np
from scipy.sparse import linalg
# 对 k 矩阵进行调节,以避免出现 "因子完全奇异" 错误
k += sparse.diags(np.random.random(k.shape[0])/1E20, shape=k.shape)
# Solve
w, v = linalg.eigsh(k, k=20, M=m, sigma=10000)
# 系统自然频率
f = (np.real(w))**0.5/(2*np.pi)
print('前四阶固有频率')
for i in range(4):
print('{:.3f} Hz'.format(f[i]))
前四阶固有频率
1283.200 Hz
1283.200 Hz
5781.975 Hz
6919.399 Hz
绘制模态振型#
您还可以绘制该有限元模型的模态振型。由于受约束的自由度已从解法中移除,因此在显示位移时必须考虑这些自由度。
import pyvista as pv
# 获取 4 阶模态振型
full_mode_shape = v[:, 3] # 每个节点的 x、y、z 位移
# 重塑并计算归一化位移
disp = full_mode_shape.reshape((-1, 3))
n = (disp*disp).sum(1)**0.5
n /= n.max() # 将数组 n 中的每个元素都除以最大值,这样 n 中的所有元素都会在 0 到 1 之间,称之为 normalize 归一化。
# 加载归档文件并创建 vtk 非结构化网格
archive = pymapdl_reader.Archive(examples.hexarchivefile)
grid = archive.parse_vtk()
# 绘制归一化位移图
# grid.plot(scalars=n)
# 绘制位移曲线
pl = pv.Plotter()
# add the nominal mesh
pl.add_mesh(grid, style='wireframe')
# copy the mesh and displace it
new_grid = grid.copy()
new_grid.points += disp/80
pl.add_mesh(new_grid, scalars=n, stitle='Normalized\nDisplacement',
flipscalars=True)
pl.add_text('Cantliver Beam 4th Mode Shape at {:.4f}'.format(f[3]),
fontsize=30)
pl.plot()
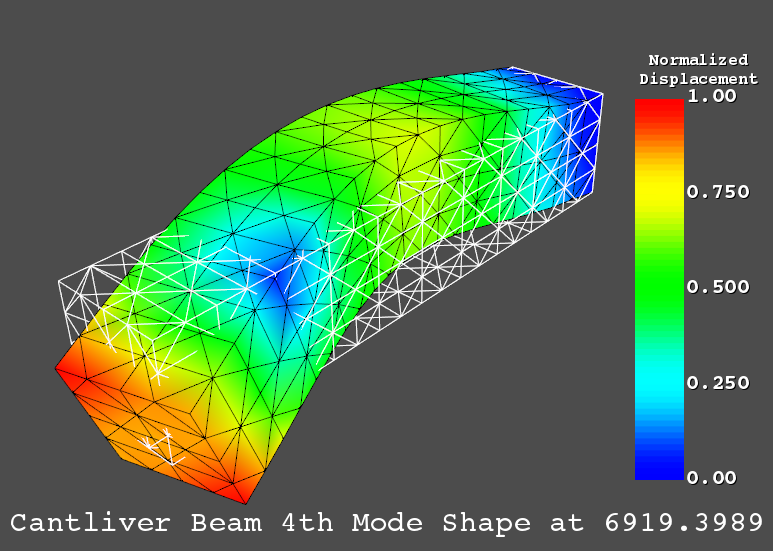
此示例内置于 pyansys-mapdl
,可通过 examples.solve_km()
运行。
FullFile 对象方法#
- class ansys.mapdl.reader.full.FullFile(filename: str | Path)#
Stores the results of an ANSYS full file.
- Parameters:
filename (str) – Filename of the full file to read.
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> full = pymapdl_reader.read_binary('file.rst') >>> print(full) PyMAPDL-Reader : MAPDL Full File Title : Demo Version : 20.1 Platform : WINDOWS x64 Jobname : file Matrices : 2 Equations : 345 Nodes : 115 Degrees of Freedom : 3
- property const#
Constrained DOF Returns the node number and DOF constrained in ANSYS.
Examples
>>> full.const array([], shape=(0, 2), dtype=int32)
- property dof_ref#
Sorted degree of freedom reference.
Examples
>>> full.dof_ref array([[ 1, 0], [ 1, 1], [ 1, 2], [115, 0], [115, 1], [115, 2]], dtype=int32)
Notes
Obtain the unsorted degree of freedom reference with >>> dof_ref, k, m = sparse_full.load_km(sort=False)
- property filename#
String form of the filename. This property is read-only.
- property k#
Stiffness Matrix corresponding to sorted DOF.
Examples
>>> from ansys.mapdl import reader as pymapdl_reader >>> full = pymapdl_reader.read_binary('file.rst') >>> print(full.k) <345x345 sparse matrix of type '<class 'numpy.float64'>' with 7002 stored elements in Compressed Sparse Column format>
- load_km(as_sparse=True, sort=False)#
Load and construct mass and stiffness matrices from an ANSYS full file.
- Parameters:
as_sparse (bool, optional) – Outputs the mass and stiffness matrices as scipy csc sparse arrays when True by default.
sort (bool, optional) – Rearranges the k and m matrices such that the rows correspond to to the sorted rows and columns in dor_ref. Also sorts dor_ref.
- Returns:
dof_ref ((n x 2) np.int32 array) – This array contains the node and degree corresponding to each row and column in the mass and stiffness matrices. In a 3 DOF analysis the dof integers will correspond to: 0 - x 1 - y 2 - z Sort these values by node number and DOF by enabling the sort parameter.
k ((n x n) np.float_ or scipy.csc array) – Stiffness array
m ((n x n) np.float_ or scipy.csc array) – Mass array
Examples
>>> dof_ref, k, m = full.load_km() >>> print(k) (0, 0) 163408119.6581276 (0, 1) 0.0423270 (1, 1) 163408119.6581276 : : (342, 344) 6590544.8717949 (343, 344) -6590544.8717950 (344, 344) 20426014.9572689
Notes
Constrained entries are removed from the mass and stiffness matrices.
Constrained DOF can be accessed from
const
, which returns the node number and DOF constrained in ANSYS.
- property load_vector#
The load vector
Examples
>>> full.load_vector array([0., 0., 0., ..., 0., 0., 0.])
- property m#
Mass Matrix corresponding to sorted DOF.
Examples
>>> full.m <345x345 sparse matrix of type '<class 'numpy.float64'>' with 2883 stored elements in Compressed Sparse Column format>
- property neqn#
Number of equations
Examples
>>> full.neqn 963
- property pathlib_filename#
Return the
pathlib.Path
version of the filename. This property can not be set.