Note
Go to the end to download the full example code
PyMAPDL 和 MAPDL 中的路径插值操作#
本教程展示了如何使用 pyansys 和 MAPDL 沿路径进行应力插值。它展示了 pyvista 模块执行插值的一些高级功能。
首先,将 MAPDL 作为服务启动,并禁用除错误信息之外的所有功能。
import matplotlib.pyplot as plt
import numpy as np
import pyvista as pv
from ansys.mapdl.core import launch_mapdl
mapdl = launch_mapdl(loglevel="ERROR")
C:\Users\ff\AppData\Local\Programs\Python\Python310\lib\site-packages\ansys\tools\path\path.py:818: DeprecationWarning: This method is going to be deprecated in future versions. Please use 'get_mapdl_path'.
warnings.warn(
MAPDL: 非均匀载荷作用下的梁#
创建梁,施加载荷,并求解静态问题。 beam dimensions
width_ = 0.5
height_ = 2
length_ = 10
# simple 3D beam
mapdl.clear()
mapdl.prep7()
mapdl.mp("EX", 1, 70000)
mapdl.mp("NUXY", 1, 0.3)
mapdl.csys(0)
mapdl.blc4(0, 0, 0.5, 2, length_)
mapdl.et(1, "SOLID186")
mapdl.type(1)
mapdl.keyopt(1, 2, 1)
mapdl.desize("", 100)
mapdl.vmesh("ALL")
# mapdl.eplot()
# fixed constraint
mapdl.nsel("s", "loc", "z", 0)
mapdl.d("all", "ux", 0)
mapdl.d("all", "uy", 0)
mapdl.d("all", "uz", 0)
# arbitrary non-uniform load
mapdl.nsel("s", "loc", "z", length_)
mapdl.f("all", "fz", 1)
mapdl.f("all", "fy", 10)
mapdl.nsel("r", "loc", "y", 0)
mapdl.f("all", "fx", 10)
mapdl.allsel()
mapdl.run("/solu")
sol_output = mapdl.solve()
# plot the normalized global displacement
mapdl.post_processing.plot_nodal_displacement(lighting=False, show_edges=True)
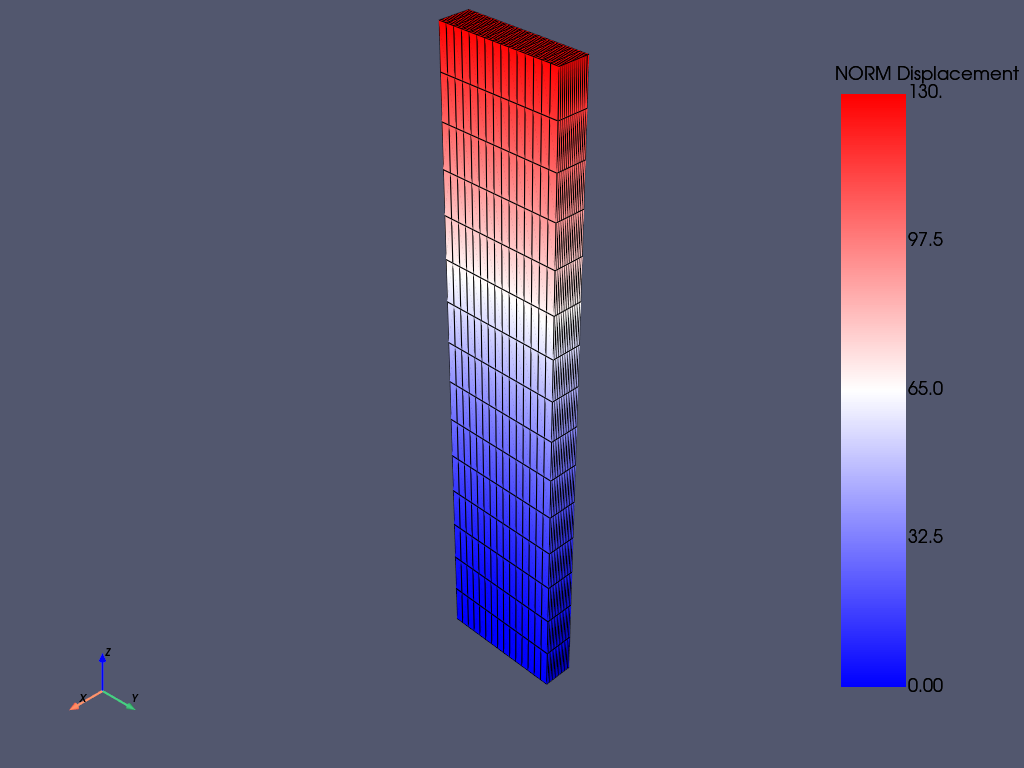
C:\Users\ff\AppData\Local\Programs\Python\Python310\lib\site-packages\wslink\backends\aiohttp\__init__.py:76: NotAppKeyWarning: It is recommended to use web.AppKey instances for keys.
https://docs.aiohttp.org/en/stable/web_advanced.html#application-s-config
self.app["state"] = {}
Post-Processing - MAPDL Path Operation#
在 MAPDL 中计算路径上的应力,并将结果转换为 numpy 数组
mapdl.post1()
mapdl.set(1, 1)
# mapdl.plesol("s", "int")
# path definition
pl_end = (0.5 * width_, height_, 0.5 * length_)
pl_start = (0.5 * width_, 0, 0.5 * length_)
mapdl.run("width_ = %f" % width_)
mapdl.run("height_ = %f" % height_)
mapdl.run("length_ = %f" % length_)
# 这些命令将 Python 变量 width_、height_ 和 length_ 的值赋给 MAPDL 中的同名变量。
# %f 是一个格式化字符串,用于将浮点数插入到字符串中。
mapdl.run("pl_end = node(0.5*width_, height_, 0.5*length_)")
mapdl.run("pl_start = node(0.5*width_, 0, 0.5*length_)")
mapdl.path("my_path", 2, ndiv=100)
mapdl.ppath(1, "pl_start")
mapdl.ppath(2, "pl_end")
# 将感兴趣的组件映射到路径上。
mapdl.pdef("Sx_my_path", "s", "x")
mapdl.pdef("Sy_my_path", "s", "y")
mapdl.pdef("Sz_my_path", "s", "z")
mapdl.pdef("Sxy_my_path", "s", "xy")
mapdl.pdef("Syz_my_path", "s", "yz")
mapdl.pdef("Szx_my_path", "s", "xz")
# 从 MAPDL 提取路径结果并发送至 numpy 数组
nsigfig = 10
# 调用 mapdl 对象的 header 方法,关闭所有的头部信息。在 ANSYS MAPDL 中,头部信息通常包括日期、时间、标题等,这里全部关闭了。
mapdl.header("OFF", "OFF", "OFF", "OFF", "OFF", "OFF")
# 调用 mapdl 对象的 format 方法,设置输出格式。这里设置的是科学计数法("E"),总宽度为 nsigfig + 9,小数部分的位数为 nsigfig。
mapdl.format("", "E", nsigfig + 9, nsigfig)
# 调用 mapdl 对象的 page 方法,设置输出页面的大小。这里设置的是每页 1e9 行,每行 240 字符。
mapdl.page(1e9, "", -1, 240)
path_out = mapdl.prpath(
"Sx_my_path",
"Sy_my_path",
"Sz_my_path",
"Sxy_my_path",
"Syz_my_path",
"Szx_my_path",
)
table = np.genfromtxt(path_out.splitlines()[1:])
print("Numpy Array from MAPDL Shape:", table.shape)
print(table)
Numpy Array from MAPDL Shape: (101, 7)
[[ 0.00000000e+00 -6.32953644e-02 -2.36286163e-01 1.09895976e+05
-1.31792517e-02 1.10710601e+02 2.67230023e+03]
[ 2.00027776e-02 -5.89959508e-02 -2.19805184e-01 1.07712769e+05
-1.21582968e-01 5.32958265e+02 2.48928469e+03]
[ 4.00055552e-02 -5.44420294e-02 -2.02923189e-01 1.05529562e+05
-2.29946191e-01 9.54657481e+02 2.30631088e+03]
[ 6.00083327e-02 -4.96336003e-02 -1.85640178e-01 1.03346356e+05
-3.38268920e-01 1.37580825e+03 2.12337880e+03]
[ 8.00111103e-02 -4.45706634e-02 -1.67956152e-01 1.01163151e+05
-4.46551154e-01 1.79641057e+03 1.94048844e+03]
[ 1.00013888e-01 -3.92532188e-02 -1.49871110e-01 9.89799461e+04
-5.54792894e-01 2.21646445e+03 1.75763981e+03]
[ 1.20016665e-01 -3.36812665e-02 -1.31385052e-01 9.67967420e+04
-6.62994140e-01 2.63596988e+03 1.57483291e+03]
[ 1.40019443e-01 -2.75263367e-02 -1.16662920e-01 9.46135409e+04
-7.42293409e-01 3.03505994e+03 1.42422517e+03]
[ 1.60022221e-01 -2.06514630e-02 -1.09654823e-01 9.24303449e+04
-7.63864078e-01 3.39403387e+03 1.33795285e+03]
[ 1.80024998e-01 -1.38093363e-02 -1.01923460e-01 9.02471490e+04
-7.85446546e-01 3.75270860e+03 1.25169049e+03]
[ 2.00027776e-01 -6.99995661e-03 -9.34688314e-02 8.80639531e+04
-8.07040812e-01 4.11108414e+03 1.16543809e+03]
[ 2.20030553e-01 -2.23323995e-04 -8.42909371e-02 8.58807572e+04
-8.28646878e-01 4.46916048e+03 1.07919565e+03]
[ 2.40033331e-01 6.52056158e-03 -7.43897770e-02 8.36975614e+04
-8.50264742e-01 4.82693762e+03 9.92963166e+02]
[ 2.60036109e-01 1.32317001e-02 -6.37653513e-02 8.15143656e+04
-8.71894405e-01 5.18441557e+03 9.06740642e+02]
[ 2.80038886e-01 1.65765453e-02 -6.52014858e-02 7.93311709e+04
-8.76703791e-01 5.49895816e+03 8.52838338e+02]
[ 3.00041664e-01 1.82404348e-02 -7.22642267e-02 7.71479766e+04
-8.73102894e-01 5.79204210e+03 8.15094373e+02]
[ 3.20044441e-01 1.98938546e-02 -7.85532530e-02 7.49647821e+04
-8.69506546e-01 6.08501719e+03 7.77352264e+02]
[ 3.40047219e-01 2.15368046e-02 -8.40685647e-02 7.27815874e+04
-8.65914747e-01 6.37788343e+03 7.39612010e+02]
[ 3.60049997e-01 2.31692849e-02 -8.88101617e-02 7.05983926e+04
-8.62327496e-01 6.67064082e+03 7.01873612e+02]
[ 3.80052774e-01 2.47912955e-02 -9.27780440e-02 6.84151976e+04
-8.58744794e-01 6.96328936e+03 6.64137070e+02]
[ 4.00055552e-01 2.64028364e-02 -9.59722117e-02 6.62320024e+04
-8.55166640e-01 7.25582905e+03 6.26402384e+02]
[ 4.20058329e-01 2.47870361e-02 -1.04626710e-01 6.40488037e+04
-8.36292382e-01 7.48840072e+03 6.10036202e+02]
[ 4.40061107e-01 2.31695758e-02 -1.12557483e-01 6.18656046e+04
-8.17418356e-01 7.72095083e+03 5.93670373e+02]
[ 4.60063885e-01 2.15504557e-02 -1.19764532e-01 5.96824054e+04
-7.98544561e-01 7.95347939e+03 5.77304896e+02]
[ 4.80066662e-01 1.99296757e-02 -1.26247856e-01 5.74992059e+04
-7.79670999e-01 8.18598638e+03 5.60939772e+02]
[ 5.00069440e-01 1.83072358e-02 -1.32007455e-01 5.53160062e+04
-7.60797668e-01 8.41847180e+03 5.44575000e+02]
[ 5.20072217e-01 1.66831360e-02 -1.37043330e-01 5.31328063e+04
-7.41924569e-01 8.65093567e+03 5.28210581e+02]
[ 5.40074995e-01 1.53782416e-02 -1.40622327e-01 5.09496072e+04
-7.19933658e-01 8.86361317e+03 5.14935780e+02]
[ 5.60077772e-01 1.47149349e-02 -1.42092169e-01 4.87664099e+04
-6.91706457e-01 9.03676485e+03 5.07839671e+02]
[ 5.80080550e-01 1.40522436e-02 -1.42959597e-01 4.65832124e+04
-6.63478840e-01 9.20993302e+03 5.00743628e+02]
[ 6.00083328e-01 1.33901676e-02 -1.43224610e-01 4.44000147e+04
-6.35250807e-01 9.38311765e+03 4.93647651e+02]
[ 6.20086105e-01 1.27287071e-02 -1.42887209e-01 4.22168168e+04
-6.07022358e-01 9.55631877e+03 4.86551740e+02]
[ 6.40088883e-01 1.20678618e-02 -1.41947394e-01 4.00336187e+04
-5.78793492e-01 9.72953635e+03 4.79455896e+02]
[ 6.60091660e-01 1.14076320e-02 -1.40405164e-01 3.78504203e+04
-5.50564211e-01 9.90277041e+03 4.72360118e+02]
[ 6.80094438e-01 1.05292745e-02 -1.36941371e-01 3.56672205e+04
-5.18695501e-01 1.00366835e+04 4.67922870e+02]
[ 7.00097216e-01 9.54191056e-03 -1.32362938e-01 3.34840197e+04
-4.85007074e-01 1.01509580e+04 4.64814876e+02]
[ 7.20099993e-01 8.55486173e-03 -1.27358910e-01 3.13008189e+04
-4.51318474e-01 1.02652656e+04 4.61706895e+02]
[ 7.40102771e-01 7.56812797e-03 -1.21929286e-01 2.91176178e+04
-4.17629702e-01 1.03796062e+04 4.58598928e+02]
[ 7.60105548e-01 6.58170927e-03 -1.16074066e-01 2.69344166e+04
-3.83940758e-01 1.04939798e+04 4.55490974e+02]
[ 7.80108326e-01 5.59560564e-03 -1.09793251e-01 2.47512152e+04
-3.50251643e-01 1.06083864e+04 4.52383034e+02]
[ 8.00111103e-01 4.60981708e-03 -1.03086840e-01 2.25680137e+04
-3.16562355e-01 1.07228259e+04 4.49275108e+02]
[ 8.20113881e-01 4.09670249e-03 -9.36630029e-02 2.03848121e+04
-2.80177140e-01 1.07783866e+04 4.47840506e+02]
[ 8.40116659e-01 3.58382009e-03 -8.40227033e-02 1.82016105e+04
-2.43791761e-01 1.08339876e+04 4.46405907e+02]
[ 8.60119436e-01 3.07116988e-03 -7.41659408e-02 1.60184088e+04
-2.07406217e-01 1.08896289e+04 4.44971312e+02]
[ 8.80122214e-01 2.55875187e-03 -6.40927155e-02 1.38352069e+04
-1.71020509e-01 1.09453106e+04 4.43536720e+02]
[ 9.00124991e-01 2.04656604e-03 -5.38030273e-02 1.16520050e+04
-1.34634637e-01 1.10010326e+04 4.42102132e+02]
[ 9.20127769e-01 1.53461240e-03 -4.32968761e-02 9.46880294e+03
-9.82485998e-02 1.10567950e+04 4.40667547e+02]
[ 9.40130547e-01 1.06425735e-03 -3.23388469e-02 7.28560084e+03
-6.16997308e-02 1.10929694e+04 4.39434554e+02]
[ 9.60133324e-01 6.76767088e-04 -2.08428561e-02 5.10239875e+03
-2.48254724e-02 1.10899296e+04 4.38604740e+02]
[ 9.80136102e-01 2.89358745e-04 -9.35440017e-03 2.91919664e+03
1.20487848e-02 1.10869339e+04 4.37774929e+02]
[ 1.00013888e+00 -9.79676843e-05 2.12652085e-03 7.35994515e+02
4.89230409e-02 1.10839822e+04 4.36945120e+02]
[ 1.02014166e+00 -4.85212200e-04 1.35999070e-02 -1.44720763e+03
8.57972958e-02 1.10810745e+04 4.36115312e+02]
[ 1.04014443e+00 -8.72374803e-04 2.50657582e-02 -3.63040980e+03
1.22671550e-01 1.10782108e+04 4.35285507e+02]
[ 1.06014721e+00 -1.25945549e-03 3.65240745e-02 -5.81361198e+03
1.59545802e-01 1.10753912e+04 4.34455703e+02]
[ 1.08014999e+00 -1.72238182e-03 4.74281754e-02 -7.99681408e+03
1.95383373e-01 1.10333660e+04 4.33618577e+02]
[ 1.10015277e+00 -2.22334303e-03 5.78659473e-02 -1.01800161e+04
2.30702465e-01 1.09717624e+04 4.32777793e+02]
[ 1.12015555e+00 -2.72440595e-03 6.80736396e-02 -1.23632180e+04
2.66021390e-01 1.09102055e+04 4.31937011e+02]
[ 1.14015832e+00 -3.22557059e-03 7.80512525e-02 -1.45464199e+04
3.01340148e-01 1.08486953e+04 4.31096233e+02]
[ 1.16016110e+00 -3.72683693e-03 8.77987858e-02 -1.67296217e+04
3.36658740e-01 1.07872319e+04 4.30255458e+02]
[ 1.18016388e+00 -4.22820499e-03 9.73162397e-02 -1.89128235e+04
3.71977165e-01 1.07258152e+04 4.29414687e+02]
[ 1.20016666e+00 -4.72967476e-03 1.06603614e-01 -2.10960252e+04
4.07295424e-01 1.06644452e+04 4.28573919e+02]
[ 1.22016943e+00 -5.68590024e-03 1.13191626e-01 -2.32792267e+04
4.38942479e-01 1.05442641e+04 4.27101127e+02]
[ 1.24017221e+00 -6.64231037e-03 1.19344663e-01 -2.54624280e+04
4.70589362e-01 1.04241335e+04 4.25628348e+02]
[ 1.26017499e+00 -7.59890516e-03 1.25062726e-01 -2.76456293e+04
5.02236072e-01 1.03040534e+04 4.24155582e+02]
[ 1.28017776e+00 -8.55568461e-03 1.30345814e-01 -2.98288304e+04
5.33882611e-01 1.01840239e+04 4.22682831e+02]
[ 1.30018054e+00 -9.51264870e-03 1.35193928e-01 -3.20120313e+04
5.65528977e-01 1.00640448e+04 4.21210092e+02]
[ 1.32018332e+00 -1.04697975e-02 1.39607067e-01 -3.41952322e+04
5.97175171e-01 9.94411629e+03 4.19737368e+02]
[ 1.34018610e+00 -1.13140044e-02 1.42918440e-01 -3.63784321e+04
6.26737319e-01 9.80463528e+03 4.17691629e+02]
[ 1.36018888e+00 -1.19322342e-02 1.44347877e-01 -3.85616304e+04
6.52131391e-01 9.62600390e+03 4.14499883e+02]
[ 1.38019165e+00 -1.25507855e-02 1.45172268e-01 -4.07448286e+04
6.77525055e-01 9.44743063e+03 4.11308203e+02]
[ 1.40019443e+00 -1.31696582e-02 1.45391614e-01 -4.29280265e+04
7.02918310e-01 9.26891549e+03 4.08116589e+02]
[ 1.42019721e+00 -1.37888523e-02 1.45005914e-01 -4.51112243e+04
7.28311156e-01 9.09045847e+03 4.04925041e+02]
[ 1.44019999e+00 -1.44083679e-02 1.44015170e-01 -4.72944219e+04
7.53703593e-01 8.91205958e+03 4.01733560e+02]
[ 1.46020276e+00 -1.50282049e-02 1.42419380e-01 -4.94776193e+04
7.79095621e-01 8.73371881e+03 3.98542145e+02]
[ 1.48020554e+00 -1.62708175e-02 1.38797942e-01 -5.16608184e+04
7.97862441e-01 8.51633474e+03 3.92632550e+02]
[ 1.50020832e+00 -1.78235578e-02 1.33766618e-01 -5.38440182e+04
8.13316989e-01 8.27947229e+03 3.85364137e+02]
[ 1.52021110e+00 -1.93749143e-02 1.28016801e-01 -5.60272178e+04
8.28771772e-01 8.04268499e+03 3.78096077e+02]
[ 1.54021387e+00 -2.09248871e-02 1.21548491e-01 -5.82104173e+04
8.44226791e-01 7.80597285e+03 3.70828369e+02]
[ 1.56021665e+00 -2.24734761e-02 1.14361687e-01 -6.03936165e+04
8.59682044e-01 7.56933587e+03 3.63561014e+02]
[ 1.58021943e+00 -2.40206815e-02 1.06456390e-01 -6.25768155e+04
8.75137533e-01 7.33277405e+03 3.56294011e+02]
[ 1.60022221e+00 -2.55665031e-02 9.78325996e-02 -6.47600144e+04
8.90593257e-01 7.09628738e+03 3.49027361e+02]
[ 1.62022498e+00 -2.39148766e-02 9.47333087e-02 -6.69432095e+04
8.90482634e-01 6.80188399e+03 3.32350474e+02]
[ 1.64022776e+00 -2.22534510e-02 9.08738701e-02 -6.91264045e+04
8.90376474e-01 6.50759453e+03 3.15675442e+02]
[ 1.66023054e+00 -2.05822262e-02 8.62542837e-02 -7.13095994e+04
8.90274778e-01 6.21341901e+03 2.99002267e+02]
[ 1.68023332e+00 -1.89012023e-02 8.08745496e-02 -7.34927941e+04
8.90177545e-01 5.91935742e+03 2.82330947e+02]
[ 1.70023609e+00 -1.72103792e-02 7.47346677e-02 -7.56759887e+04
8.90084775e-01 5.62540978e+03 2.65661482e+02]
[ 1.72023887e+00 -1.55097570e-02 6.78346380e-02 -7.78591831e+04
8.89996469e-01 5.33157608e+03 2.48993874e+02]
[ 1.74024165e+00 -1.22010489e-02 6.62971862e-02 -8.00423781e+04
8.81974482e-01 5.01820044e+03 2.25256125e+02]
[ 1.76024443e+00 -5.66882837e-03 7.62758436e-02 -8.22255742e+04
8.58085358e-01 4.66568319e+03 1.87381641e+02]
[ 1.78024720e+00 8.98900934e-04 8.55405618e-02 -8.44087704e+04
8.34207733e-01 4.31336421e+03 1.49517115e+02]
[ 1.80024998e+00 7.50213901e-03 9.40913408e-02 -8.65919666e+04
8.10341607e-01 3.96124348e+03 1.11662549e+02]
[ 1.82025276e+00 1.41408859e-02 1.01928181e-01 -8.87751629e+04
7.86486979e-01 3.60932100e+03 7.38179407e+01]
[ 1.84025554e+00 2.08151415e-02 1.09051081e-01 -9.09583592e+04
7.62643850e-01 3.25759678e+03 3.59832912e+01]
[ 1.86025832e+00 2.75249059e-02 1.15460043e-01 -9.31415556e+04
7.38812220e-01 2.90607082e+03 -1.84139956e+00]
[ 1.88026109e+00 3.35392183e-02 1.30250108e-01 -9.53247563e+04
6.61929725e-01 2.52019796e+03 -6.74205704e+01]
[ 1.90026387e+00 3.89770459e-02 1.49147709e-01 -9.75079596e+04
5.58485087e-01 2.11734270e+03 -1.46845528e+02]
[ 1.92026665e+00 4.41545668e-02 1.67660117e-01 -9.96911635e+04
4.55000996e-01 1.71479598e+03 -2.26228757e+02]
[ 1.94026943e+00 4.90717807e-02 1.85787333e-01 -1.01874368e+05
3.51477453e-01 1.31255780e+03 -3.05570259e+02]
[ 1.96027220e+00 5.37286878e-02 2.03529354e-01 -1.04057573e+05
2.47914457e-01 9.10628169e+02 -3.84870033e+02]
[ 1.98027498e+00 5.81252881e-02 2.20886183e-01 -1.06240778e+05
1.44312009e-01 5.09007075e+02 -4.64128080e+02]
[ 2.00027776e+00 6.22615814e-02 2.37857819e-01 -1.08423984e+05
4.06701077e-02 1.07694524e+02 -5.43344399e+02]]
Comparing with Path Operation Within pyvista#
同样的路径操作也可以在 pyvista 中执行,方法是保存生成的应力并存储在底层的 UnstructuredGrid 中。
请注意,MAPDL 和 VTK 的插值方法(两者的插值结果几乎相同)都有轻微的分段行为。VTK 的基本算法是:
Note
一旦找到包含查询点的单元格, vtkProbeFilter 就会使用单元格的插值函数来执行插值/计算点的属性。
# same thing in pyvista
rst = mapdl.result
nnum, stress = rst.nodal_stress(0)
# get SYZ stress
stress_yz = stress[:, 5] # 第 5 列
# 将 YZ 应力分配给结果实例中的底层网格。
# 在本例中,NAN 值必须替换为 0,插值才能成功
stress_yz[np.isnan(stress_yz)] = 0
rst.grid["Stress YZ"] = stress_yz
# 创建一条线并在其上取样
line = pv.Line(pl_start, pl_end, resolution=100)
out = line.sample(rst.grid) # bug where the interpolation must be run twice
out = line.sample(rst.grid)
# 注:我们本可以使用样条曲线(或任何数据集),并在其上进行插值,而不是简单的直线。
# 绘制 VTK 和 MAPDL 的内插应力图
plt.plot(out.points[:, 1], out["Stress YZ"], "x", label="Stress vtk")
plt.plot(table[:, 0], table[:, 6], label="Stress MAPDL")
plt.legend()
plt.xlabel("Y Position (in.)")
plt.ylabel("Stress YZ (psi)")
plt.show()
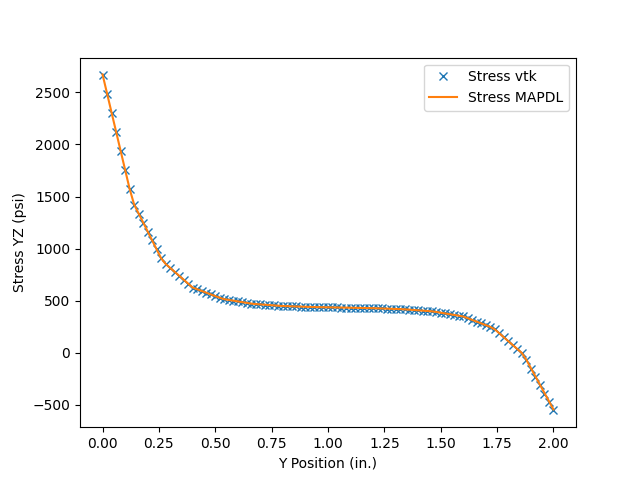
2D Slice Interpolation#
沿梁绘制二维切片,并将其与该线上的应力一并绘制。
请注意,该切片发生在该梁的边缘节点之间,由于应力/应变(通常)会外推到 ANSYS 有限元的边缘节点,因此有必要进行插值。
stress_slice = rst.grid.slice("z", pl_start)
# 可以单独绘制
# stress_slice.plot(scalars=stress_slice['Stress YZ'],
# scalar_bar_args={'title': 'Stress YZ'})
# 良好的摄像机位置(使用 pl.camera_position 手动确定)
cpos = [(3.2, 4, 8), (0.25, 1.0, 5.0), (0.0, 0.0, 1.0)]
max_ = np.max((out["Stress YZ"].max(), stress_slice["Stress YZ"].max()))
min_ = np.min((out["Stress YZ"].min(), stress_slice["Stress YZ"].min()))
clim = [min_, max_]
pl = pv.Plotter()
pl.add_mesh(
out,
scalars=out["Stress YZ"],
line_width=10,
clim=clim,
scalar_bar_args={"title": "Stress YZ"},
)
pl.add_mesh(
stress_slice,
scalars="Stress YZ",
opacity=0.25,
clim=clim,
show_scalar_bar=False,
)
pl.add_mesh(rst.grid, color="w", style="wireframe", show_scalar_bar=False)
pl.camera_position = cpos
_ = pl.show()
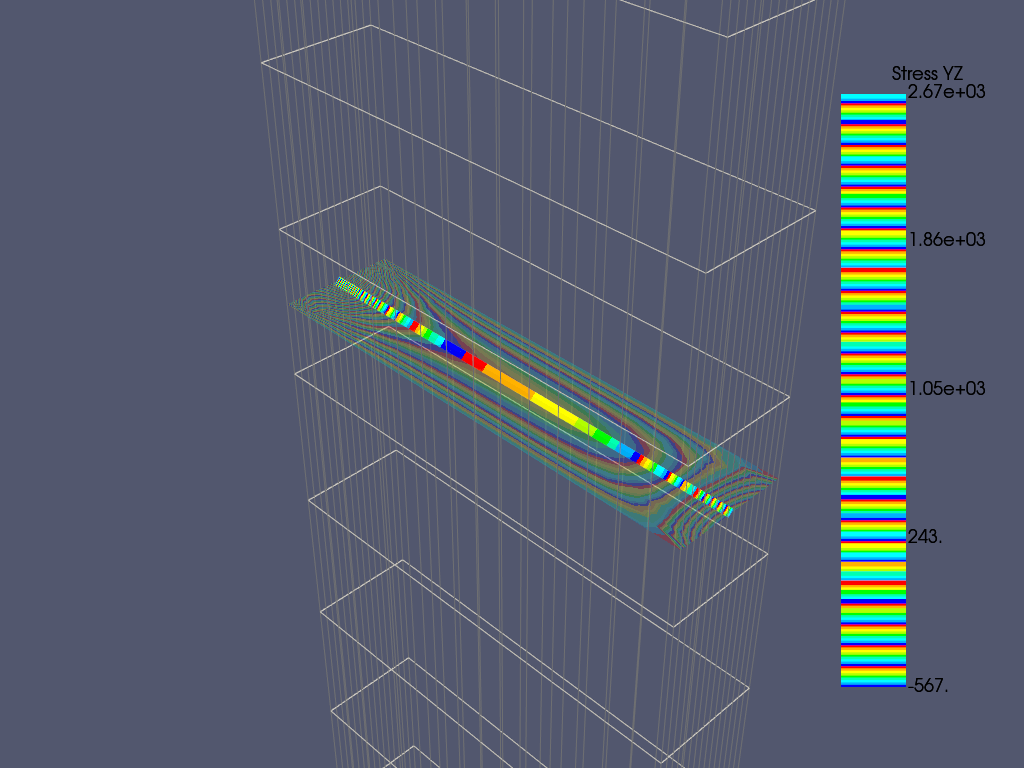
Stop mapdl#
mapdl.exit()
Total running time of the script: (0 minutes 12.732 seconds)